MapReduce序列化案例
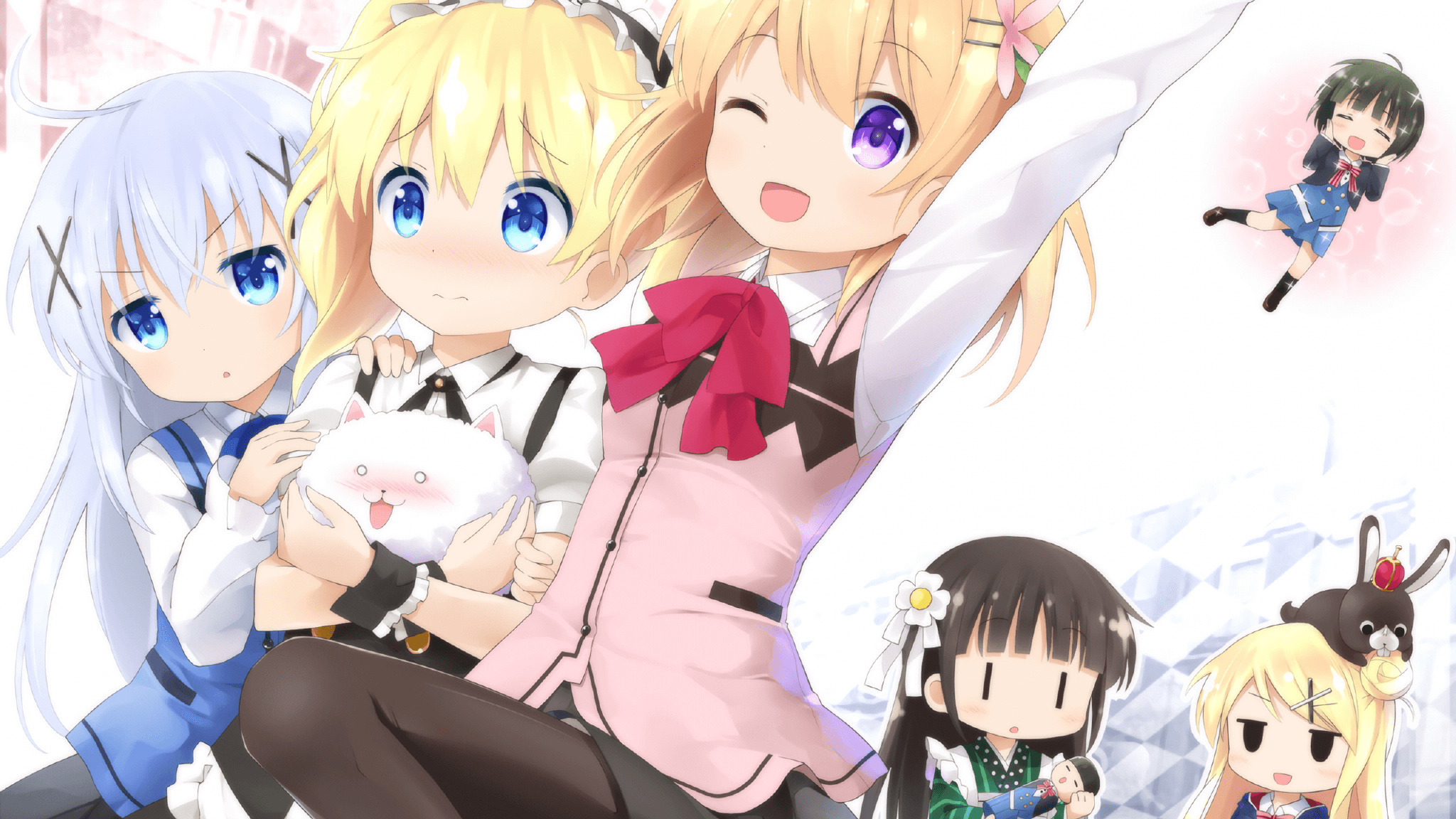
MapReduce序列化案例
小贾嗯嗯输入数据
1 | id 手机号 ip 网址 上行流量 下行流量 请求状态 |
FlowBean
1 | package flowsum; |
FlowCountMapper
1 | package flowsum; |
FlowCountReducer
1 | package flowsum; |
FlowCountDriver
1 | package flowsum; |
结果
1 | 13004073160 1494 75 1569 |
评论
匿名评论隐私政策
1 | id 手机号 ip 网址 上行流量 下行流量 请求状态 |
1 | package flowsum; |
1 | package flowsum; |
1 | package flowsum; |
1 | package flowsum; |
1 | 13004073160 1494 75 1569 |